In this article, we will learn about displaying data in react that is in the form of array data structures. Arrays are the most popular and efficient data structures that are used to store data. We will learn to display linear data stored in an array in multiple ways.
The data in the arrays can be used by using the numerical index of the array item in the pattern array sequence works. In simple words, we can say that arrays are list-like objects with various methods that can be used to traverse the array and also methods for performing operations on the arrays such as sorting, filtering, removing and adding items, and many more.
Let’s take an example to understand a real-world example for understanding various scenarios of using arrays if you are using them in your projects. Almost all real-world projects use tables to store data and consider what tables generally are collections of rows and columns. So, we could consider a table as a two-dimensional array, where every element of the array is every cell of the table.
Example:
items = [
[“grapes”, “apple”],
[“orange”, “watermelon”],
[“Mango”, “strawberry”]
];

Two-dimensional array
As seen in an example of the array above, two-dimensional arrays are arrays in which each element is another array. In simple language, we can say that two-dimensional arrays are an array of arrays.
To properly understand the concept of two-dimensional arrays, let’s use developer tools for it. Using the array “items” that we declared as an example above in the console. Using the console.table() function to display our data in the items array, we get the proper visualization of our data in the form of a table where the column index indicates the number of rows starting with zero, column “0” indicated the first elements of the arrays of our array item and the column “1” indicated the second element of our arrays of the array
Creating Two-dimensional array
Now we already have an idea about what a two-dimensional array is and what it should look like. Let’s try to create a two-dimensional array using some methods that we can use for mutations of the arrays.
Example:
let matrix = Array(3).fill(0).map((row,index) =>
new Array(3).fill('Row ' + (index+1))
)
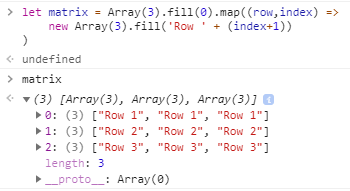
The fill() method changes all elements in an array to a static value, from a start index (default 0) to an end index (default array.length). It returns the modified array. Referred from online documents. In the example above we will create an array of length three, fill it with all the zeros, and replace every element with another array of three elements.
Demo
Array data in the form of Table
We will read earlier that the best example of two-dimensional data is a table. So let’s first try to display a two-dimensional array in the form of a table. Here we will use a simple HTML table, but you can also use some UI libraries to display the data.
Example array:
fruits = [
["Fruit", "Quantity"],
["Apple", 80],
["Orange", 70],
["watermelon", 50],
["Mango", 100]
];
We will use various array methods to display the data of the array in a tabular manner. Some of the functions such as map and slice. Let’s first have a look at the example after that we will understand the use of the methods that will use.
import React from "react";
import "./style.css";
const DataInTable = () => {
const fruits = [
["Fruit", "Quantity"],
["Apple", 80],
["Orange", 70],
["watermelon", 50],
["Mango", 100]
];
return (
<div>
<table className="dataintable">
<thead>
<tr>
{fruits[0].map((item, index) => {
return (
<th className="tableHead" key={"head" + index}>
{item}
</th>
);
})}
</tr>
</thead>
<tbody>
{fruits.slice(1, fruits.length).map((item, index) => {
return (
<tr key={"row-" + index}>
<td>{item[0]}</td>
<td>{item[1]}</td>
<td>{item[2]}</td>
</tr>
);
})}
</tbody>
</table>
</div>
);
};
export default DataInTable;
DataInTable.js
The first row of our is used for displaying the headings. We will use the map function to traverse the elements to display in our table head cells. We have used fruits[0] as we are sure that we will have the table headings in the first row.
After that, we used the slice function of the array where the rows other than the heading will be extracted using the function. After getting the rest of the data from the array, we will traverse through the array so that we can display the in our HTML table.
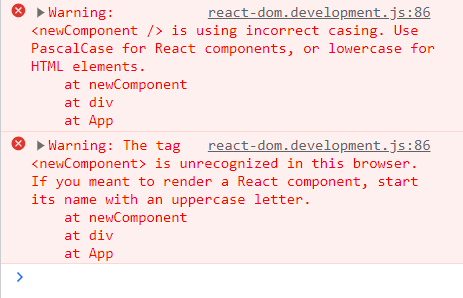
When displaying in tabular form the output will be as shown in the image above.
Array in the form of a List
In the above section, we use the two-dimensional array to display the data in tabular format. Two-dimensional data can be also used to display the data in the form of a list. The list can either be an ordered list or an unordered list. In the above section, we used the map function to traverse through the data, we will be using the same in this approach. We can also use for loop to traverse through all the elements of the array.
When we displayed the data in tabular form, we used one map function to traverse through elements to display the heading of the table and used another map function to traverse through the rest of the elements of the array.
Example array:
items = [
[“grapes”, “apple”, “kiwi”],
[“orange”, “watermelon”],
[“Mango”, “strawberry”]
];
To display the elements as a list we will use a simple HTML unordered list. We will use the map function, that we have already used. We will create a separate functional component for implementing the data as a list.
import React from "react";
const DataAsList = () => {
const fruits = [
["grapes", "apple", "kiwi"],
["orange", "watermelon"],
["Mango", "strawberry"]
];
return (
<div>
{fruits.map((items, index) => {
return (
<ul key={"ul-" + index}>
{items.map((subItems, sIndex) => {
return <li key={"li-" + sIndex}> {subItems} </li>;
})}
</ul>
);
})}
</div>
);
};
export default DataAsList;
As seen in the code above, we have declared a two-dimensional array named fruits. The first map function traverses through the arrays in the fruit array. The second map function traverses through the elements of the arrays within the array. In simple words, first, we will traverse through the fruits array, so that we will get an individual item as the inner array, now we will traverse the items using another map function.

When displaying the data as a list, the output would be something as shown in the image above.
Things to keep in mind
Always add a key attribute when using the map function. As we know that react re-rendering works when anything in the virtual DOM is updated. The virtual DOM is used to apply the new changes in the actual DOM using a diffing algorithm with a unique value (key) to identify the modifications in the virtual DOM elements.
In the code above I have already added the keys for dynamic rows or list items that are being generated so we won’t get any warnings, but if we forget to include the keys then we will get a warning to use a key value for the dynamically generated row elements.
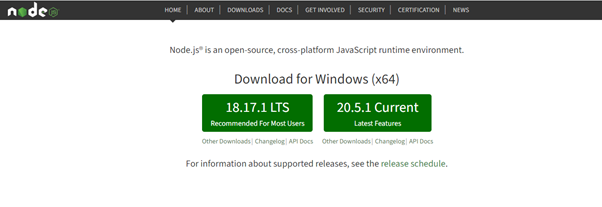
Demo
Summary
In this article, we learned about displaying the data from a two-dimensional array. We also learned what is two-dimensional array be having a look at some examples. We used the array functions such as map and slice. After reading this article we have a clear idea of using the array data and displaying it in various formats.